Watchdog Timer: The Secret to Reliable System Operation
When it comes to designing embedded systems and other applications where uptime and reliability are critical, a watchdog timer can be a powerful tool. In this post, we’ll take a closer look at what watchdog timers are, how they work, and why they’re so important for reliable system operation.
Content
What is a Watchdog Timer?
At its core, a watchdog timer is a hardware or software component that is designed to detect and recover from system malfunctions. It works by monitoring the system and triggering a reset if a failure is detected. The goal is to ensure that the system always operates within a certain set of parameters and doesn’t drift off course due to unexpected events or bugs.
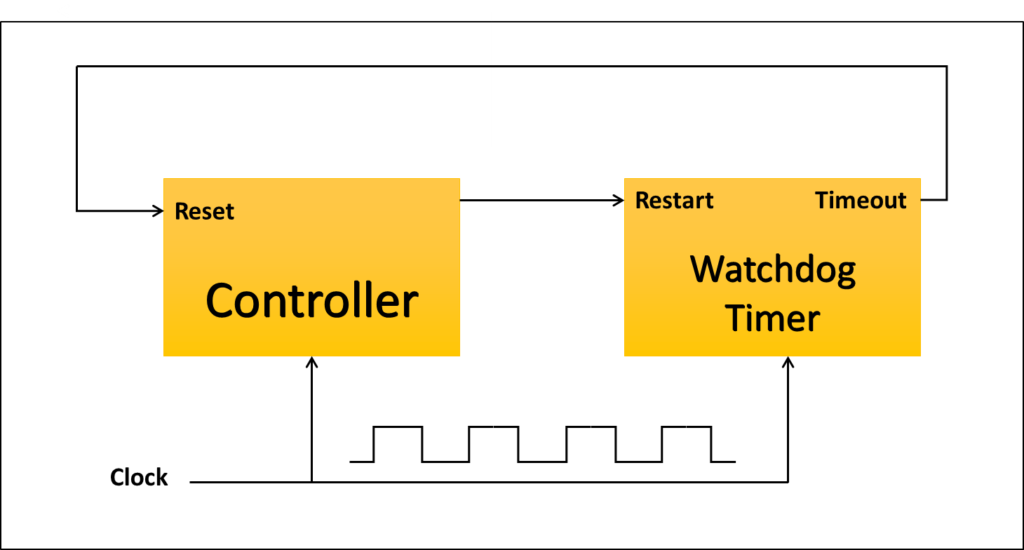
How Does a Watchdog Timer Work?
A watchdog timer works by periodically monitoring the system and resetting it if a certain condition is met. The exact implementation can vary depending on the specific system and application, but here’s a high-level overview of how it typically works:
- The watchdog timer is initialized with a timeout value. This value determines how long the watchdog timer will wait before triggering a reset.
- The system or application being monitored regularly resets the watchdog timer. This can be done in a variety of ways, such as writing to a specific memory location or sending a signal.
- If the watchdog timer is not reset within the specified timeout period, it assumes that a failure has occurred and triggers a reset of the system or application.
- The reset caused by the watchdog timer will typically be a “hard” reset, which means that the system or application will be completely restarted from scratch.
Why are Watchdog Timers Important?
So why are watchdog timers so important for reliable system operation? There are a few key reasons:
- Automatic recovery: By using a watchdog timer, it’s possible to automatically recover from software or hardware failures without requiring human intervention. This can help to improve the overall reliability and availability of a system or application.
- Preventative maintenance: By regularly resetting the watchdog timer, the system can proactively identify and correct potential issues before they become major problems.
- Real-time monitoring: A watchdog timer can provide real-time monitoring of a system or application, giving engineers insight into its performance and behavior.
- Compliance: For some industries, such as aerospace and defense, watchdog timers are a mandatory requirement to ensure that systems operate within certain safety-critical parameters.
Types of Watchdog Timers
There are two main types of watchdog timers: hardware and software.
Hardware watchdog timers are typically built into a microcontroller or other hardware device. They use a dedicated hardware counter to track the timeout period and trigger a reset when necessary.
Software watchdog timers, on the other hand, are implemented in software using a timer interrupt. They use the system clock to track the timeout period and trigger a reset when necessary.
Implementing a Watchdog Timer
Implementing a watchdog timer requires careful consideration of the system’s requirements and architecture. Some key factors to consider include the timeout value, the reset mechanism, and the system’s power consumption.
The timeout value should be chosen to be longer than the maximum expected time between system resets, but not so long that a malfunction could go undetected for an extended period. The reset mechanism should be designed to bring the system back to a known state, such as restarting the application or reinitializing key components. The system’s power consumption should also be considered, as a watchdog timer that is constantly running can consume significant power over time.
// Define the timeout value (in milliseconds)
const int WATCHDOG_TIMEOUT = 5000;
// Define the function to reset the watchdog timer
void resetWatchdogTimer() {
// Write a value to a specific memory location or send a signal to reset the watchdog timer
}
// Initialize the watchdog timer
void initWatchdogTimer() {
// Set up the timer hardware or software interrupt to trigger every WATCHDOG_TIMEOUT milliseconds
}
// Main loop
void mainLoop() {
// Reset the watchdog timer at the beginning of the loop
resetWatchdogTimer();
// Do some work...
// Check for any errors or unexpected conditions
if (errorDetected()) {
// Take corrective action...
// Reset the watchdog timer again
resetWatchdogTimer();
}
}
// Watchdog timer interrupt handler
void watchdogTimerInterruptHandler() {
// If this function is called, it means that the watchdog timer has timed out
// Take appropriate action, such as resetting the system or application
}
Applications of Watchdog Timers
Watchdog timers are commonly used in safety-critical applications such as aerospace, automotive, and medical devices. They are also used in industrial control systems, embedded systems, and other applications where system uptime and reliability are critical.
In safety-critical applications, watchdog timers are often required by industry standards and regulations to ensure that systems operate within certain safety-critical parameters. For example, in the aerospace industry, watchdog timers are used to ensure that flight control systems operate safely and reliably.
Conclusion
Overall, a watchdog timer can be a powerful tool for ensuring reliable system operation in a wide range of applications. By carefully selecting the timeout value and implementing a proper reset mechanism, it’s possible to design systems that are highly resilient and always operate within expected parameters. Whether you’re designing a safety-critical system or just want to ensure that your application runs reliably, a watchdog timer is a tool that should be in every designer’s toolkit.
Read My Other Blogs:
Embedded C language Interview Questions.
Embedded System Interview Questions.
Automotive Interview Questions