C is a general-purpose programming language that was created in the early 1970s by Dennis Ritchie at Bell Labs. It has since become one of the most widely used programming languages and has influenced many other languages.
C Coding Interview Questions are generally asked in interview.
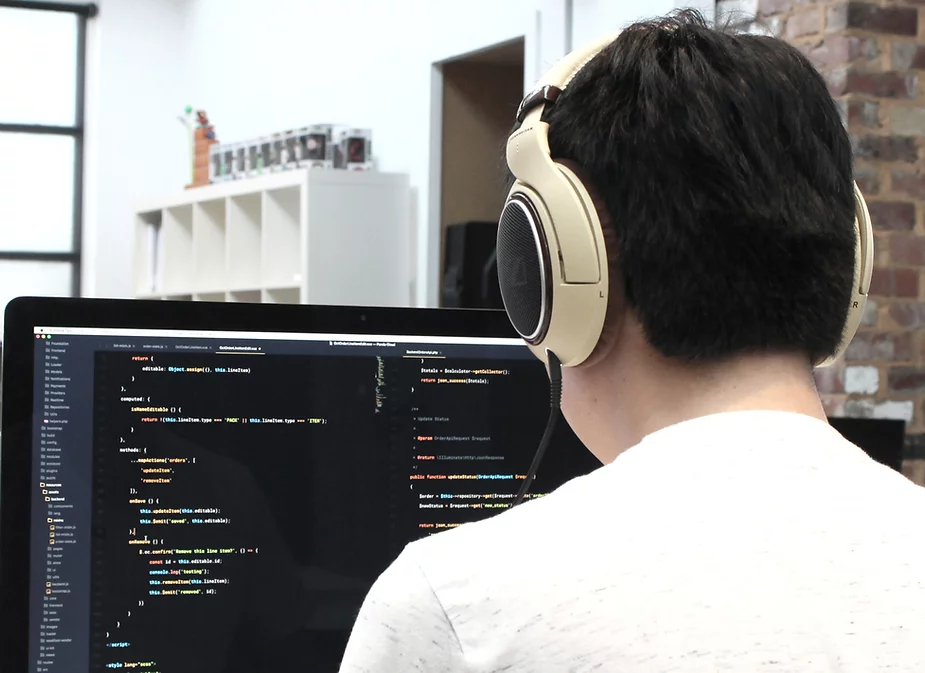
Index
1) Write a Program in C to Swap the values of two variables without using any extra variable.
#include <stdio.h>
int main() {
int a, b;
// Input values for variables a and b
printf("Enter value for variable 'a': ");
scanf("%d", &a);
printf("Enter value for variable 'b': ");
scanf("%d", &b);
// Swapping without using extra variable
a = a + b;
b = a - b;
a = a - b;
// Output the swapped values
printf("After swapping:\n");
printf("Value of variable 'a': %d\n", a);
printf("Value of variable 'b': %d\n", b);
return 0;
}
Youtube: https://www.youtube.com/watch?v=CUyWLHh7_e4
2) Write a Program to convert the binary number into a decimal number.
#include <stdio.h>
int binaryToDecimal(int binaryNumber) {
int decimalNumber = 0, base = 1, remainder;
while (binaryNumber > 0) {
remainder = binaryNumber % 10;
decimalNumber += remainder * base;
binaryNumber /= 10;
base *= 2;
}
return decimalNumber;
}
int main() {
int binaryNumber;
// Input binary number
printf("Enter a binary number: ");
scanf("%d", &binaryNumber);
// Convert binary to decimal
int decimalNumber = binaryToDecimal(binaryNumber);
// Output the result
printf("Decimal equivalent: %d\n", decimalNumber);
return 0;
}
3) Write a Program to reverse a number.
#include <stdio.h>
int reverseNumber(int num) {
int reversed = 0;
while (num != 0) {
int digit = num % 10;
reversed = reversed * 10 + digit;
num /= 10;
}
return reversed;
}
int main() {
int num;
// Input number
printf("Enter a number: ");
scanf("%d", &num);
// Reverse the number
int reversedNum = reverseNumber(num);
// Output the result
printf("Reversed number: %d\n", reversedNum);
return 0;
}
4) Write a C Program to check if two numbers are equal without using the bitwise operator.
#include <stdio.h>
int main() {
int num1, num2;
// Input numbers
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
// Check if the numbers are equal without bitwise operators
if (num1 - num2 == 0) {
printf("The numbers are equal.\n");
} else {
printf("The numbers are not equal.\n");
}
return 0;
}
5) Write a C Program to find the Maximum and minimum of two numbers without using any loop or condition.
#include <stdio.h>
int main() {
int num1, num2;
// Input numbers
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
// Find maximum without using conditional statements
int max = num1 - ((num1 - num2) & ((num1 - num2) >> (sizeof(int) * 8 - 1)));
// Find minimum without using conditional statements
int min = num2 + ((num1 - num2) & ((num1 - num2) >> (sizeof(int) * 8 - 1)));
// Output the results
printf("Maximum of %d and %d is: %d\n", num1, num2, max);
printf("Minimum of %d and %d is: %d\n", num1, num2, min);
return 0;
}
6) Write a program to reverse an Array.
#include <stdio.h>
void reverseArray(int arr[], int size) {
int start = 0;
int end = size - 1;
while (start < end) {
// Swap elements at start and end indices
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
// Move indices towards the center
start++;
end--;
}
}
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
int size;
// Input the size of the array
printf("Enter the size of the array: ");
scanf("%d", &size);
int arr[size];
// Input elements of the array
printf("Enter %d elements of the array:\n", size);
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Reverse the array
reverseArray(arr, size);
// Output the reversed array
printf("Reversed array:\n");
printArray(arr, size);
return 0;
}
7) Write a C Program to search elements in an array.
#include <stdio.h>
int searchElement(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return i; // Return the index if the element is found
}
}
return -1; // Return -1 if the element is not found
}
int main() {
int size, target;
// Input the size of the array
printf("Enter the size of the array: ");
scanf("%d", &size);
int arr[size];
// Input elements of the array
printf("Enter %d elements of the array:\n", size);
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Input the element to search
printf("Enter the element to search: ");
scanf("%d", &target);
// Search for the element in the array
int index = searchElement(arr, size, target);
// Output the result
if (index != -1) {
printf("Element %d found at index %d.\n", target, index);
} else {
printf("Element %d not found in the array.\n", target);
}
return 0;
}
8) Write a C Program to sort arrays using Bubble, Selection, and Insertion Sort.
#include <stdio.h>
// Function to perform Bubble Sort on an array
void bubbleSort(int arr[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
// Swap elements if they are in the wrong order
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// Function to perform Selection Sort on an array
void selectionSort(int arr[], int size) {
for (int i = 0; i < size - 1; i++) {
int minIndex = i;
// Find the minimum element in the unsorted part of the array
for (int j = i + 1; j < size; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// Swap the found minimum element with the first element
int temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
// Function to perform Insertion Sort on an array
void insertionSort(int arr[], int size) {
for (int i = 1; i < size; i++) {
int key = arr[i];
int j = i - 1;
// Move elements of arr[0..i-1] that are greater than key to one position ahead of their current position
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
// Function to print the elements of an array
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
int size;
// Input the size of the array
printf("Enter the size of the array: ");
scanf("%d", &size);
int arr[size];
// Input elements of the array
printf("Enter %d elements of the array:\n", size);
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Perform Bubble Sort and print the sorted array
printf("\nBubble Sort:\n");
bubbleSort(arr, size);
printArray(arr, size);
// Reset the array for Selection Sort
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Perform Selection Sort and print the sorted array
printf("\nSelection Sort:\n");
selectionSort(arr, size);
printArray(arr, size);
// Reset the array for Insertion Sort
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Perform Insertion Sort and print the sorted array
printf("\nInsertion Sort:\n");
insertionSort(arr, size);
printArray(arr, size);
return 0;
}
9) Write a Program to Replace all 0’s with 1’s in a Number.
#include <stdio.h>
int replaceZerosWithOnes(int num) {
int result = 0, power = 1;
while (num > 0) {
int digit = num % 10;
// Replace 0 with 1
if (digit == 0) {
result += 1 * power;
} else {
result += digit * power;
}
power *= 10;
num /= 10;
}
return result;
}
int main() {
int number;
// Input the number
printf("Enter a number: ");
scanf("%d", &number);
// Replace 0's with 1's
int result = replaceZerosWithOnes(number);
// Output the result
printf("Number after replacing 0's with 1's: %d\n", result)
return 0;
}
10) Write a program to check the repeating elements in C.
#include <stdio.h>
void findRepeatingElements(int arr[], int size) {
printf("Repeating elements in the array are: ");
for (int i = 0; i < size; i++) {
for (int j = i + 1; j < size; j++) {
if (arr[i] == arr[j]) {
printf("%d ", arr[i]);
break; // Once a repeating element is found, break out of the inner loop
}
}
}
printf("\n");
}
int main() {
int size;
// Input the size of the array
printf("Enter the size of the array: ");
scanf("%d", &size);
int arr[size];
// Input elements of the array
printf("Enter %d elements of the array:\n", size);
for (int i = 0; i < size; i++) {
scanf("%d", &arr[i]);
}
// Find and print repeating elements
findRepeatingElements(arr, size);
return 0;
}
Read my other blogs:
C Program to find Given Number is Prime or not.
Write a program to find Factorial Numbers of a given numbers.
Embedded C language Interview Questions.
Automotive Interview Questions
Understanding AUTOSAR Architecture: A Guide to Automotive Software Integration
Big Endian and Little Endian in Memory
Zero to Hero in C language Playlist
Embedded C Interview Questions
Subscribe my channel on Youtube: Yogin Savani