Bit manipulation in C language. In this blog, we will see How to Set, Clear, and Toggle a bit in C language using the Bitwise operator.
Content:
we need 3 things. 1) Number 2) Position and 3) Output.
Setting a bit. :
Ex: Number is 10.
The binary of 10 is 1010 -> 2nd bit is 0 need to set with 1. so my output will be 14 (1110).

If the bit is already set then do nothing and the output should be as it is.

how to reach at 2nd Position?
we will use the left shift operator and reach position. (1<<pos).
then we do a Bitwise OR operation with a number. num | (1<<pos) then the number became set.
num= num | (1<<pos) // Set a bit
Clear a Bit
Ex: Number is 10
The binary of 10 is 1010 -> 3rd bit is 1 need to Clear 0. so my output will be 2 (0110).
for that I need to reach position first using shift operator (1<< pos). then do a compliment.
that output will do Bitwise AND Operation with Number.
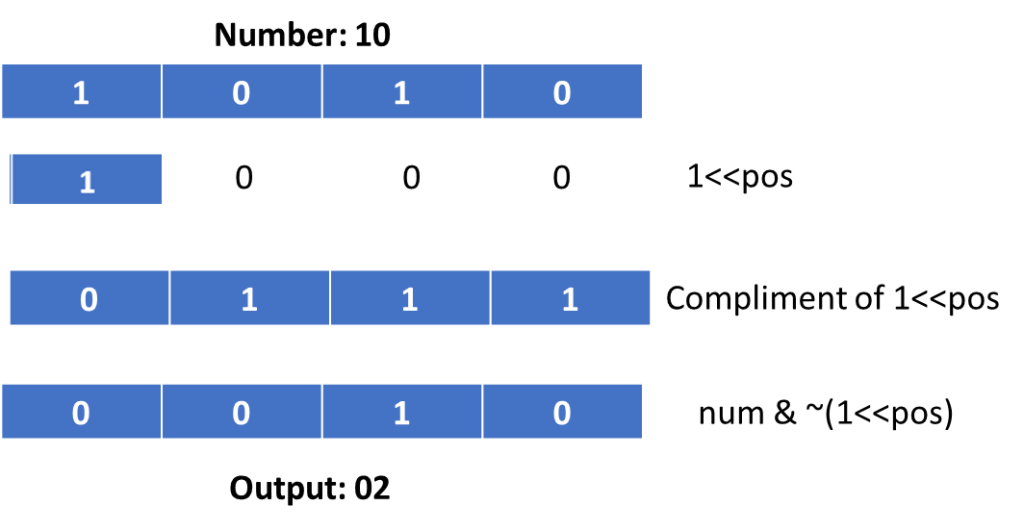
num= num & ~(1<<pos) // Clear a bit
Toggle (Compliment) a bit
Ex: Number is 10
The binary of 10 is 1010 -> 2nd bit is 0 need to set 1. so my output will be 14 (1110).
or Binary of 14(1110) -> 2nd bit is 1 need to clear 0. so my output will be 10 (1010).

we will use the left shift operator and reach position. (1<<pos).
then we do a Bitwise EXOR operation with a number. num ^ (1<<pos) then the number became a compliment If it is 0 then became 1 or If it is 0 then became 1.
num= num ^ (1<<pos) // Toggle a bit
How to Find Particular bit is Set or clear.
We need to check particular bit is set or clear. not want to do any perform set clear and toggle bit.
Here our output is True (1) or False (0).
Example:
The number is 10 want to check if the 2nd position is set or clear.

res= num & (1<<pos) or
res= (num >>pos) & 1)
Summary
Set a bit : num= num | (1<<pos)
Clear a bit : num= num & ~(1<<pos)
Toggle a bit: num= num ^ (1<<pos)
Particular bit set or clear: res= num & (1<<pos) or res= (num >>pos) & 1)
Watch the Below Video to get a Complete understanding with programming.
Read my other blogs:
C Program to find Given Number is Prime or not.
Write a program to find Factorial Numbers of a given numbers.
Embedded C language Interview Questions.
Automotive Interview Questions
Understanding AUTOSAR Architecture: A Guide to Automotive Software Integration
Big Endian and Little Endian in Memory
Zero to Hero in C language Playlist
Embedded C Interview Questions
Subscribe my channel on Youtube: Yogin Savani