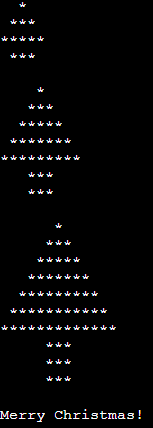
As the festive season approaches, spreading joy and cheer becomes a delightful tradition. One creative way to celebrate is by crafting a simple C language program that wishes “Merry Christmas” through a festive pattern. In this blog post, we’ll guide you through the process of creating a Christmas tree using C.
Creating the Christmas Tree
Let’s begin by creating a simple Christmas tree pattern. We’ll use a combination of loops to draw the tree. Open your C file (e.g., merry_christmas.c
) and start with the following code:
#include <stdio.h>
void printSpaces(int n) {
for (int i = 0; i < n; i++) {
printf(" ");
}
}
void printStars(int n) {
for (int i = 0; i < n; i++) {
printf("*");
}
}
void printTree(int rows) {
int space = rows - 1;
// Print the tree pattern
for (int i = 1; i <= rows; i++) {
printSpaces(space);
printStars(2 * i - 1);
printf("\n");
space--;
}
}
void printTrunk(int rows) {
for (int i = 0; i < rows / 2; i++) {
printSpaces(rows - 2);
printStars(3);
printf("\n");
}
}
int main() {
int treeRows = 3; // Number of layers in the Christmas tree
// Loop for each layer
for (int layer = 0; layer < treeRows; layer++) {
// Calculate rows for each layer
int rows = 2 * (layer + 1) + 1;
// Print the Christmas tree for the current layer
printTree(rows);
// Print the trunk of the tree for the current layer
printTrunk(rows);
// Add some space between layers
printf("\n");
}
// Print the Christmas greeting
printf("Merry Christmas!\n");
return 0;
}
In this program, I’ve added a loop to control the number of layers in the Christmas tree (treeRows
). Each layer consists of a tree pattern and a trunk. The printTree
and printTrunk
functions are responsible for printing the patterns for the tree and trunk, respectively. Adjust the value of treeRows
to control the number of layers in the Christmas tree.
Read my other blogs:
C Program to find Given Number is Prime or not.
Write a program to find Factorial Numbers of a given numbers.
Embedded C language Interview Questions.
Automotive Interview Questions
Understanding AUTOSAR Architecture: A Guide to Automotive Software Integration
Big Endian and Little Endian in Memory
Zero to Hero in C language Playlist
Embedded C Interview Questions
Subscribe my channel on Youtube: Yogin Savani