In the world of programming, pointers are a fundamental concept, particularly in languages like C, C++, and similar low-level or system-level programming languages. Among these, the null pointer plays a crucial role in ensuring the reliability and safety of applications. This blog will delve into what a null pointer is, why it’s important, common issues associated with it, and best practices for handling it.
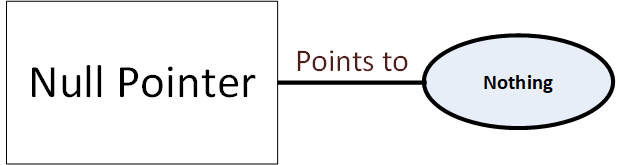
What is a Null Pointer?
A null pointer is a pointer that does not point to any valid memory address or object. It is used to signify that the pointer is not currently assigned or is intentionally invalid. In C and C++, a null pointer is often represented using the keyword NULL
or the nullptr
constant (introduced in C++11).
Representation of Null Pointer
In C, a null pointer is often defined as:
#define NULL ((void*)0)
In C++, nullptr
is preferred over NULL
because it is type-safe and avoids ambiguity in function overloading.
Example of Null Pointer
#include <stdio.h>
int main() {
int *ptr = NULL; // ptr is a null pointer
if (ptr == NULL) {
printf("Pointer is null.\n");
}
return 0;
}
Why Null Pointers are Important
Null pointers serve several critical purposes in programming:
- Indicator of Uninitialized or Invalid Pointers:
- Null pointers help signal that a pointer does not reference any object or memory location, reducing the risk of accidental access to invalid memory.
- Default Initialization:
- Assigning a null pointer as the default ensures safety when a pointer’s value is not yet known.
- Error Handling:
- Functions that return pointers can use a null pointer to indicate an error or special condition.
- Dynamic Memory Management:
- Null pointers are commonly used in dynamic memory allocation to check whether memory allocation was successful or not.
Null Pointer vs. Void Pointer
Aspect | Null Pointer | Void Pointer |
---|---|---|
Definition | A pointer that points to nothing. | A generic pointer that can hold the address of any type. |
Purpose | Used to represent an uninitialized or invalid pointer. | Used for type-agnostic pointer operations. |
Type-Specific | Null pointers are type-specific. | Void pointers are type-independent. |
Example | int *ptr = NULL; | void *vptr; |
Common Issues with Null Pointers
While null pointers are useful, improper handling can lead to significant issues:
1. Null Pointer Dereference
Dereferencing a null pointer is undefined behavior and often leads to program crashes or segmentation faults.
int *ptr = NULL;
*ptr = 10; // Undefined behavior
2. Accidental Null Pointer Access
Sometimes, logic errors in a program can result in an attempt to access or modify data through a null pointer.
3. Difficult Debugging
Null pointer errors can be hard to debug, especially in large codebases, because they often manifest as crashes without clear error messages.
Best Practices for Handling Null Pointers
To avoid issues with null pointers, programmers should follow best practices:
1. Explicitly Initialize Pointers
Always initialize pointers to a known value, preferably NULL
or nullptr
.
int *ptr = NULL;
2. Check for Null Before Dereferencing
Always check if a pointer is null before attempting to dereference it.
if (ptr != NULL) {
*ptr = 10;
}
3. Avoid Mixing Raw Pointers and Null Checks
Using nullptr
(C++11 and later) instead of NULL
ensures type safety and avoids ambiguity in function calls.
// cpp
void func(int *ptr) {}
func(nullptr); // Preferred over func(NULL);
4. Enable Compiler Warnings
Modern compilers can warn about potential null pointer dereferences. Enable these warnings and address them promptly.
5. Adopt Static Analysis Tools
Static analysis tools can detect null pointer dereference risks during development.
Real-World Applications of Null Pointers
1. Error Handling in Memory Allocation
Dynamic memory allocation functions like malloc
and calloc
return null pointers when memory allocation fails.
int *arr = (int*)malloc(10 * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed.\n");
}
2. Data Structures
Null pointers are extensively used in linked data structures such as linked lists and trees to signify the end of a structure.
struct Node {
int data;
struct Node *next;
};
Node *head = NULL; // Empty linked list
3. Optional Return Values
Functions returning pointers often use null pointers to indicate the absence of a value.
char *find_char(const char *str, char c) {
while (*str) {
if (*str == c) return (char*)str;
str++;
}
return NULL; // Character not found
}
Common Misconceptions About Null Pointers
- Null Pointer is Always Address Zero:
- While null pointers often have an address of zero, this is not guaranteed by the C standard. It depends on the hardware and compiler implementation.
- Dereferencing Null Always Crashes:
- In some systems, dereferencing a null pointer might not immediately crash but can lead to subtle bugs and undefined behavior.
Conclusion
The null pointer is an essential concept in low-level programming, offering a mechanism to handle invalid or uninitialized pointers effectively. While it introduces potential pitfalls like null pointer dereferences, these risks can be mitigated with proper coding practices, robust error handling, and modern tools like smart pointers in C++.
Understanding null pointers and managing them wisely can significantly enhance the stability and reliability of your software. Whether you are working on embedded systems, application development, or complex data structures, mastering null pointers is a critical step toward becoming a proficient programmer.